Firebase는 Google에서 제공하는 클라우드 기반 모바일 및 웹 애플리케이션 개발 플랫폼으로, 실시간 데이터베이스, 인증, 저장소, 애널리틱스, 앱푸시 등 다양한 기능을 제공합니다. 이 중에서도 앱푸시 기능은 모바일 애플리케이션 개발에 있어 중요한 기능 중 하나입니다. 이번에는 Firebase를 이용하여 앱푸시 기능을 구축하는 방법을 설명하겠습니다.
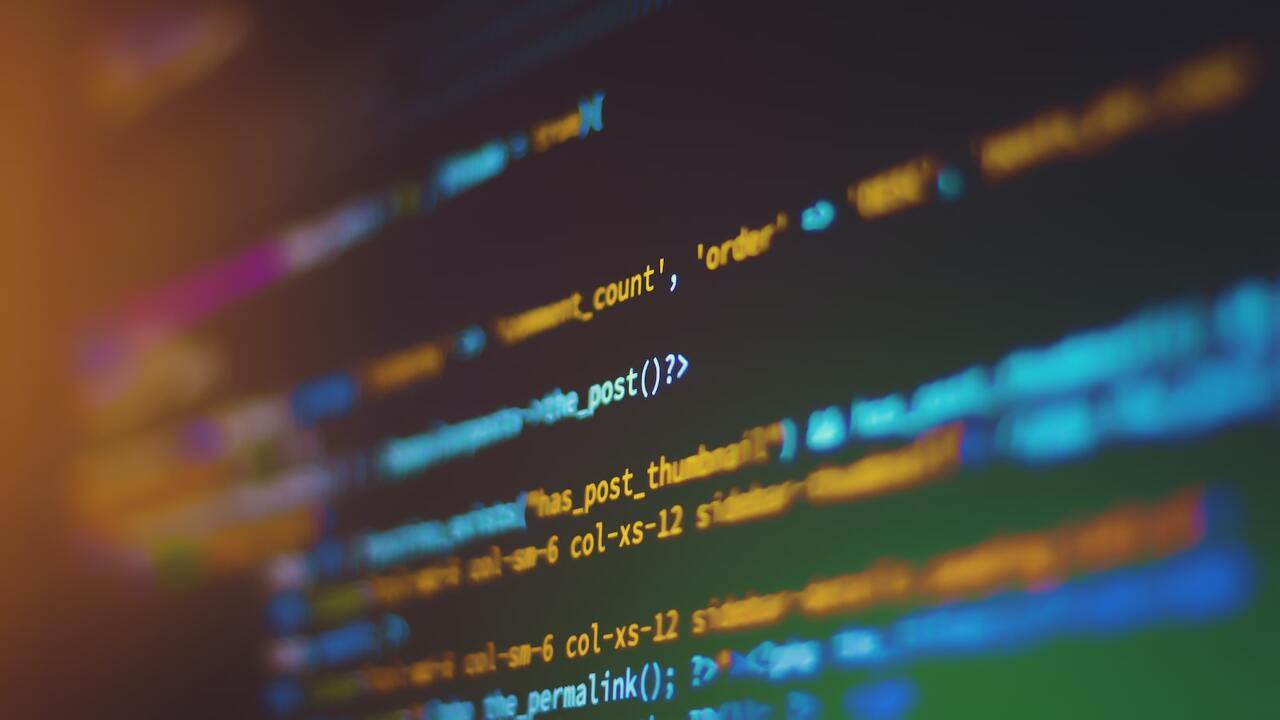
Firebase 프로젝트 생성하기
Firebase를 이용하기 위해서는 먼저 Firebase 프로젝트를 생성해야 합니다. Firebase 콘솔에 접속하여 "프로젝트 만들기" 버튼을 클릭합니다. 프로젝트 이름과 국가를 선택한 후 "프로젝트 만들기" 버튼을 클릭합니다.
Firebase SDK 추가하기
Firebase를 이용하기 위해서는 먼저 Firebase SDK를 추가해야 합니다. 프로젝트 생성이 완료되면 콘솔에서 "프로젝트 설정" 메뉴로 이동하여 "내 앱 추가" 버튼을 클릭합니다. 앱 이름을 입력한 후, 안드로이드 또는 iOS 앱을 선택하고 패키지 이름 또는 번들 ID를 입력합니다. 그리고 "앱 추가" 버튼을 클릭합니다.
앱 추가가 완료되면, "google-services.json" 파일(Android) 또는 "GoogleService-Info.plist" 파일(iOS)을 다운로드 받습니다.
FCM 설정하기
Firebase Cloud Messaging(FCM)은 Firebase에서 제공하는 앱푸시 서비스입니다. FCM을 이용하기 위해서는 FCM 설정이 필요합니다. FCM 설정을 위해 콘솔에서 "클라우드 메시징" 메뉴로 이동합니다.
안드로이드의 경우, "안드로이드 앱 등록" 버튼을 클릭하여 패키지 이름과 SHA-1 인증서 지문을 입력합니다.
iOS의 경우, "iOS 앱 등록" 버튼을 클릭하여 번들 ID와 APNs 인증서(.p8 파일)를 등록합니다.
Firebase SDK 연동하기
Android Studio를 실행하여 "build.gradle(Module: app)" 파일에 Firebase SDK를 추가합니다. 다음 코드를 파일에 추가합니다.
dependencies {
// Firebase SDK
implementation 'com.google.firebase:firebase-messaging:21.1.0'
}
iOS의 경우, Cocoapods를 이용하여 Firebase SDK를 추가합니다. "Podfile" 파일에 다음 코드를 추가한 후, "pod install" 명령어를 실행합니다.
pod 'Firebase/Core'
pod 'Firebase/Messaging'
FCM 메시지 수신하기
Firebase SDK를 연동한 후에는 FCM 메시지를 수신할 수 있습니다. 메시지를 수신하기 위해서는 FirebaseMessagingService를 상속받은 클래스를 생성합니다. 이 클래스에서는 FirebaseMessagingService 클래스의 onMessageReceived() 메서드를 오버라이드하여 메시지를 처리할 수 있습니다. 다음은 예시 코드입니다.
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
// 메시지 처리 코드 작성
}
}
안드로이드의 경우, AndroidManifest.xml 파일에 다음 코드를 추가하여 MyFirebaseMessagingService 클래스를 등록합니다.
<service
android:name=".MyFirebaseMessagingService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
iOS의 경우, AppDelegate.swift 파일에 다음 코드를 추가하여 Firebase SDK를 초기화합니다.
import Firebase
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
return true
}
}
그리고 iOS에서도 FCM 메시지를 처리하기 위해 UNUserNotificationCenterDelegate 프로토콜을 상속받은 클래스를 생성해야 합니다. 이 클래스에서는 UserNotifications.framework에서 제공하는 UNUserNotificationCenterDelegate 프로토콜의 메서드를 구현하여 메시지를 처리할 수 있습니다. 다음은 예시 코드입니다.
import Firebase
import UserNotifications
class MyNotificationDelegate: NSObject, UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
// 메시지 처리 코드 작성
}
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// 메시지 처리 코드 작성
}
}
iOS에서 FCM 메시지를 처리하기 위해서는 위에서 작성한 MyNotificationDelegate 클래스의 인스턴스를 UNUserNotificationCenter.current().delegate에 등록해야 합니다.
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
let notificationDelegate = MyNotificationDelegate()
UNUserNotificationCenter.current().delegate = notificationDelegate
return true
}
FCM 메시지 보내기
Firebase 콘솔을 이용하여 FCM 메시지를 보낼 수 있습니다. 콘솔에서 “클라우드 메시징” 메뉴로 이동한 후, “새 메시지 보내기” 버튼을 클릭합니다. 메시지를 입력한 후, “테스트 전송” 또는 “실제 전송” 버튼을 클릭합니다.
Firebase SDK를 이용하여 FCM 메시지를 보내려면, FirebaseMessaging 클래스의 send() 메서드를 호출합니다. 안드로이드의 경우, 다음은 예시 코드입니다.
FirebaseMessaging.getInstance().send(new RemoteMessage.Builder("SENDER_ID" + "@gcm.googleapis.com")
.setMessageId(Integer.toString(msgId++))
.addData("my_message", "Hello World")
.addData("my_action","SAY_HELLO")
.setToken(token)
.build());
ios의 경우, 다음은 예시 코드입니다.
let message = ["message": "Hello World"]
Messaging.messaging().send(
message,
to: "DEVICE_TOKEN"
) { error in
if let error = error {
print("Error sending message: \(error.localizedDescription)")
} else {
print("Message sent successfully")
}
}
푸시 알림 수신 허용
FCM 메시지를 수신하려면, 사용자가 푸시 알림을 수신 허용해야 합니다. 안드로이드의 경우, 사용자에게 알림 수신 권한을 요청하기 위해서는 다음과 같이 권한을 추가하고 사용자에게 권한을 요청해야 합니다.
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<application>
<service
android:name=".MyFirebaseMessagingService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
<activity
android:name=".MainActivity"
android:label="@string/app_name"
android:theme="@style/AppTheme.NoActionBar">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver
android:name=".MyFirebaseMessagingReceiver"
android:exported="true"
android:enabled="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</receiver>
<meta-data
android:name="com.google.firebase.messaging.default_notification_icon"
android:resource="@drawable/ic_notification" />
<meta-data
android:name="com.google.firebase.messaging.default_notification_color"
android:resource="@color/colorAccent" />
</application>
iOS의 경우, 사용자에게 푸시 알림을 수신할 지 물어보기 위해서는 다음과 같이 알림 권한을 요청해야 합니다.
import UserNotifications
UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .sound, .badge]) { granted, error in
if let error = error {
print("Error requesting authorization: \(error.localizedDescription)")
} else if granted {
print("Authorization granted")
} else {
print("Authorization not granted")
}
}
이렇게 하면 사용자가 앱에서 푸시 알림을 수신할 수 있게 됩니다. 위에서 작성한 FCM 메시지 보내기와 함께 사용하면, 앱에서 사용자에게 메시지를 전송할 수 있습니다.
'APP > Firebase' 카테고리의 다른 글
Firebase 서버를 구축하는 방법 (0) | 2023.02.24 |
---|
댓글